DevOps with Boto Python - Part V
Monty: The Security Guard!
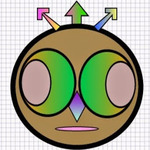
Monty: The Security Guard! Yes, In this article you will learn,
- How to list security groups
- How to create a security group
- How to apply rules to a security group
- How to revoke rules from a security group
- How to remove a security group
Here are few words about a security group.
A security group acts as a virtual firewall that controls the traffic for one or more instances. When you launch an instance, you associate one or more security groups with the instance. You add rules to each security group that allow traffic to or from its associated instances.
Now let's add a new file Security.py to our project. Just like other class files, we will place this file under the "aws" package.
Riteshs-MacBook-Pro-2:DevOps rpatel$ cd aws
Riteshs-MacBook-Pro-2:aws rpatel$ touch Security.py
Now, let's add a Security class to this file.
import boto.ec2
class Security:
def __init__(self):
''' Security Constructor '''
Modify "init.py" to add a reference of Security class.
from Connection import Connection
from EC2Instance import EC2Instance
from Volumes import Volumes
from Security import Security
Modify "DevOpsMain.py" to add a reference of Security class.
#Import classes from aws package
from aws import Connection
from aws import EC2Instance
from aws import Volumes
from aws import Security
All set!
Boto: List Security Groups
To list the security groups we will add a function list_security_groups to Security class. Each security group has (should have) distinct rules tied with it. Therefore, while printing the details on security groups we will also extract rules defined for each security group.
Let's add the list_security_groups() function.
import boto.ec2
class Security:
def __init__(self):
''' Security Constructor '''
def list_security_groups(self,conn):
''' Lists Security Groups '''
Now, let's append some code to list group name, description and the VPC Id it's attached to.
import boto.ec2
class Security:
def __init__(self):
''' Security Constructor '''
def list_security_groups(self,conn):
''' Lists Security Groups '''
groups = conn.get_all_security_groups()
if groups:
for g in groups:
print 'Group Name: ', g.name
print 'Description: ', g.description
print 'VPC Id: ', g.vpc_id
In the code above we make a call to get_all_security_groups(). It will return listing of security groups from our AWS account. Next, we loop through groups and print group name, group description and vpc id.
Next, we will print rules defined for each security group.
import boto.ec2
class Security:
def __init__(self):
''' Security Constructor '''
def list_security_groups(self,conn):
''' Lists Security Groups '''
groups = conn.get_all_security_groups()
if groups:
# loop through each group
for g in groups:
#print group name, description & vpc id
print 'Group Name: ', g.name
print 'Description: ', g.description
print 'VPC Id: ', g.vpc_id
#print rules tied with each security group
rules = g.rules
if rules:
ruleStr = ""
#loop through rules
for r in range(len(rules)):
#extract protocol, from port, to port & grants given by each rule
protocol = rules[r].ip_protocol
from_port = rules[r].from_port
to_port = rules[r].to_port
grants = rules[r].grants
if r == 0:
ruleStr = ""
else:
ruleStr = ruleStr + '\n'
if protocol:
ruleStr = ruleStr + 'Protocol: ' + protocol
if from_port:
ruleStr = ruleStr + ' | From Port: ' + from_port
if to_port:
ruleStr = ruleStr + ' | To Port: ' + to_port
if grants:
ruleStr = ruleStr + ' | Grants: ' + str(grants).strip('[]')
print ruleStr
print '****************************************'
Let's review the code again. We extract rules from the group. Then, loop through each rule and extract details of each rule. Finally we print the rule and security group information.
Now let's modify DevOpsMain.py file to make a call to list_security_groups().
#!/usr/bin/Python
#Import classes from aws package
from aws import Connection
from aws import EC2Instance
from aws import Volumes
from aws import Security
connInst = Connection()
conn = connInst.ec2Connection()
#Manage Security Groups
sgInst = Security()
sgInst.list_security_groups(conn)
As usual we are back to Python Interpreter to execute the script.
Riteshs-MacBook-Pro-2:DevOps rpatel$ python DevOpsMain.py
Protocol: -1 | Grants: sg-f1ffe123-454543866811
Protocol: tcp | From Port: 22 | To Port: 22 | Grants: 0.0.0.0/0
Protocol: -1 | Grants: 10.190.2.0/22
Protocol: tcp | From Port: 1194 | To Port: 1194 | Grants: 54.230.115.71/32
********************************************
And to my surprise I see details of one and only security group from my account. Now, of course this code should list all security groups from your account. You get the idea.
Next we will create a security group from scratch.
Boto: Add a Guard... Create a Security Group
Quite obviously we will add a new function add_security_group to Security.py class. We will also add a code to create a security group and attach some rules to the group.
import boto.ec2
class Security:
def __init__(self):
''' Security Constructor '''
def add_security_group(self,conn):
''' Creates a new Security Group '''
#create a database security group
dbsg = conn.create_security_group('RAP-DB-SG','Database Security Group')
if dbsg:
#add an ingress rule for Oracle
dbsg.authorize('tcp','1521','1521','68.255.14.150/32')
#add an ingress rule for SQL Server
dbsg.authorize('tcp','1433','1433','68.255.14.150/32')
dbsg.authorize('udp','1434','1434','68.255.14.150/32')
Let's review the code now. First we create a security group by calling create_security_group. We specify the name of our security group RAP-DB-SG and a description of the security group, which is Database Security Group.
Next, we check if the security group was created successfully. If so, we add some database rules to this security group. Because our group is a Database Security Group we will add some rules for Oracle & SQL Server. Now of course you may add as many database rules as you wish. PostgreSQL, MongoDB and so on... To add a rule we made a call to authorize with some parameters. For our rule we specified,
- a protocol
- from port
- to port
- ip address
You know how to execute the script at Python prompt. Hint: Make a call to add_security_group from DevOpsMain.py and now you should have a database security group with few rules attached to it.
Revoke access....Revoke a rule from the Security Group
In the previous section we added few rules to our Database Security Group. Now let's revoke one of the rules. Let's say we want this group to be strictly a SQL Server Group. Well, then we will have to revoke a rule for the Oracle database.
Let's add a function revoke_security_rule.
import boto.ec2
class Security:
def __init__(self):
''' Security Constructor '''
def revoke_security_rule(self,conn,sgname,ruletype):
''' Revoke a rule from the security group '''
#get a specific security group
grp = conn.get_all_security_groups(sgname)
#revoke a rule from the security group
if grp:
for g in grp:
if ruletype == 'oracle':
g.revoke('tcp',1521,1521,'68.255.14.150/32')
In the code above, we are extracting a specific security group. In our case it is RAP-DB-SG group. Next we get a handle of the security group and check which rule type has to be revoked. Finally, we call revoke to remove the rule from a security group. As you noticed, while making a call to revoke we are passing,
- a protocol
- from port
- to port
- ip address
Next let's modify the DevOpsMain.py script to revoke the rule.
#!/usr/bin/Python
#Import classes from aws package
from aws import Connection
from aws import EC2Instance
from aws import Volumes
from aws import Security
#Manage Security Groups
sgInst = Security()
sgInst.revoke_security_rule(conn,'RAP-DB-SG','oracle','12.123.123.22/32')
We modified DevOpsMain.py to make a call to revoke_security_rule. Along with we passed the name of a security group, type of rule to be revoked and the ip address.
Upon executing DevOpsMain.py at Python prompt, the script should remove a rule for Oracle database from our security group.
Easy, right?
So far we learned how to create a security group, add rules to the security group and revoke specific rules from a security group. Finally, we will see how to remove a security group.
Remove Guard...Remove a Security Group
You guessed it right! We will add a new function remove_security_group to our Security class.
import boto.ec2
class Security:
def __init__(self):
''' Security Constructor '''
def remove_security_group(self,conn,sgname):
''' Remove a security group '''
#get a specific security group
grp = conn.get_all_security_groups(sgname)
#remove security group
if grp:
for g in grp:
g.delete()
Code above is very easy to understand. We get a handle of specific security group. In our case it is RAP-DB-SG. Upon retrieving handle to the security group we call group.delete.
Next we modify DevOpsMain.py script to make a call to remove_security_group.
#!/usr/bin/Python
#Import classes from aws package
from aws import Connection
from aws import EC2Instance
from aws import Volumes
from aws import Security
#Manage Security Groups
sgInst = Security()
sgInst.remove_security_group(conn,'RAP-DB-SG')
Execute DevOpsMain.py at the Python prompt and ka..boom! Our security group is gone from the list. Forever!
Like I said: Boto is amazing. I am loving it and you will too.
Project files are located @ github. Fork and enjoy!
See you then!
Hi, I am Ritesh Patel. I live in a beautiful town surrounded by the mountains. C&O Canal is few miles away. State parks are only a distance away & bike trails galore. It is home sweet home Frederick, MD. A passionate developer. Love to cook. Enjoy playing "Bollywood Tunes" on my harmonica. Apart from that just a normal guy.