DevOps with Boto Python - Part I
Meet Monty Py!
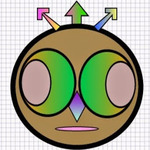
Yeah, I just found my DevOps hat and guess what? I am ready to put it on. In this tutorial series we will learn how to DevOps in Amazon Cloud using Python Boto.
First let me say this: Boto is amazing. I love it and if you are on Amazon Cloud you will love it too. I promise.
First things first. Let's look at the pre-requisites, assumptions and facts for this tutorial series.
Pre Requisites
- Python 2.7 or up
- IDE of your choice
- Boto
- AWS Secret Key & Access Id
- You must know Python. I learned it and I am sure you can too. Really cool language. Google "python tutorial" and you will find really awesome resources on learning Python. Remember, it's not about the snake!
Assumptions
- You are familiar with AWS technologies
- You are familiar with Python
Facts
- I am on a Mac OS X
- I am using Sublime Text as my IDE
- I will refer to Mac utilities throughout the series
Off we go.
Install Python
If you are on a Mac OS X, like I am then you already have a Python installed. If you wish to upgrade then you may do so. Go to the Mac terminal and type,
python --version
You should see an output similar to Python 2.7.5. An official version of Python installed on your machine.
As for Windows download the latest release and follow the instructions on installing Python.
Assuming python is installed. Next up is installing Boto.
Install & Configure Boto
Use pip to install Boto. At the terminal type,
pip install boto
Next, we will configure AWS credentials with Boto. Create a file under your user directory. At the Mac terminal type,
# go to your home directory
Riteshs-MacBook-Pro-2:CSSReport rpatel$ cd ~
# create a new file .boto
Riteshs-MacBook-Pro-2:CSSReport rpatel$ touch .boto
You should be in the vi editor with .boto file open in vi. Next, type in your AWS credentials in this file. Do you have the credentials? If not then go to AWS IAM page and generate your access key id and secret access key. Once you have the key and id add them to .boto file.
#credentials tobe saved in the .boto file
[Credentials]
aws_access_key_id = YOURACCESSKEY
aws_secret_access_key = YOURSECRETACCESSKEY
Note: It is important you type the entire text above and replace YOURACCESSKEY / YOURSECRETACCESSKEY with the actual values.
By now you should have Python and Boto configured. Let's run a simple test with Boto.
Test Boto
At the Mac terminal fire up Python.
# fire up python interpreter
Riteshs-MacBook-Pro-2:~ rpatel$ python
Python 2.7.5 (default, Mar 9 2014, 22:15:05)
[GCC 4.2.1 Compatible Apple LLVM 5.0 (clang-500.0.68)] on darwin
Type "help", "copyright", "credits" or "license" for more information.
>>>
>>> indicates that I am at the python prompt. Next we will import boto.ec2 and connect to a specific region of AWS.
# import boto EC2
>>> import boto.ec2
# connect to AWS region
>>> ec2 = boto.ec2.connect_to_region('us-east-1')
# print EC2 connection object
>>> print ec2
# terminal output of the connection object
EC2Connection:ec2.us-east-1.amazonaws.com
>>>
In the code above, I am importing boto.ec2 and making a connection to us-east-1 region of AWS. But wait, where are my credentials? Well, we saved the credentials in .boto file, remember? Python terminal will read the credentials from the file and make a connection. Once I have the connection I print the connection object. And Voila! Terminal output shows EC2Connection.
I am happy now.
Python Executable Script
We can run python commands at python prompt. But how about executing a python script? Let's look at how to create an executable file. Open up a text editor or an IDE of your choice and create a new file called "HelloMonty.py". I use Sublime Text and you should too. Believe me, it is awesome. Type the contents below in this file.
#!/usr/bin/python
print "Hello Monty Py! Glad to have met ya!"
Save the file and run the command below at the terminal.
# execute the python script
Riteshs-MacBook-Pro-2:DevOpsTut rpatel$ python HelloMonty.py
# terminal output from the script
Hello Monty Py! Glad to have met ya!
I see a friendly message: Hello Monty Py! Glad to have met ya!
Project files are located @ github. Fork and enjoy!
In the next article we will setup a simple python project to begin our DevOps adventure. What are you waiting for? Let's move on!
Hi, I am Ritesh Patel. I live in a beautiful town surrounded by the mountains. C&O Canal is few miles away. State parks are only a distance away & bike trails galore. It is home sweet home Frederick, MD. A passionate developer. Love to cook. Enjoy playing "Bollywood Tunes" on my harmonica. Apart from that just a normal guy.