Node REST API - Part I
feel like resting today :-)
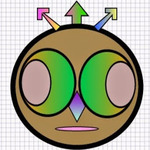
Welcome to Node REST API tutorial series. Glad to have you here. In this tutorial series I will show you how to write a functional Node REST API using Express JS framework & MongoDB.
First things first. Let’s get our development environment ready.
Install IDE
I use Sublime Text as my IDE. Isn’t it just a “text editor”? No, it’s lot more than that. Check it out @ www.sublimetext.com. Go and install a version for your operating system. Use sublime text package manager to find, install and keep packages up-to-date. You will find wide range of packages @ https://sublime.wbond.net/. Customize your IDE as you wish.
Install Node & NPM - A Node Package Manager
Download & Install Node binary from http://nodejs.org/. Node is installed now, but how do you really know it’s installed and ready to go? Well, open up a command line and type in
node --version
If Node is installed correctly then you should see a node version at command line. With Node comes a Node Package Manager (NPM). If you don’t know what is NPM, then go to https://www.npmjs.org/ and learn more about it. NPM is used to install and manage node packages. Syntax for installing a node package is as easy as npm install package-name --save. Later in the article you will see how we install “express” using npm install command. Super easy!
Install MongoDB
MongoDB is a NoSQL database. A documents database. We will be using MongoDB as a backend for our REST API. Therefore, let’s install Mongo. Go to http://docs.mongodb.org/manual/installation/ and select a binary that suits your platform. I used “manual install”. Pretty easy to set it up.
- Download Mongo binary
- Create a directory called “MongoDB” and extract binaries under that folder
- Create a “data/db” directory to hold databases. Remember, Mongo databases are simply a collection of documents.
- Modify permissions on “data/db” folder for the current user that will be running Mongo.
chmod 755 -R /data
- Add Mongo path to your environment. Open “terminal” and run following commands.
- echo “$PATH”. This will print the current path from your environment. It should look something like /usr/bin:/bin:/usr/sbin:/sbin:/usr/local/bin
- mongo -version. Since our system doesn’t know about mongo executable yet, you will see an output “mongo: command not found”. As expected. Nothing to worry about.
- cd ~ : to go to your home directory
- pwd : to see your current working directory. In my case I see /Users/rpatel.
- touch .bash_profile : Creates a .bash_profile file.
- sudo pico .bash_profile : Edit the file in a pico editor (for mac)
- export MONGO_PATH=~/mongodb: Exports a MONGO_PATH variable.
- export PATH=$PATH:$MONGO_PATH/bin : Adds mongo bin directory to the PATH.
- Restart your terminal.
- Run mongo -version : And you should see mongo version. I see “MongoDB shell version: 2.6.5”
- Finally, just to see what your PATH looks like, run : echo $PATH. Boom, it spits out your entire path. I see /usr/bin:/bin:/usr/sbin:/sbin:/usr/local/bin:/Users/rpatel/mongodb/bin
Now you have mongo running on your local machine. Next, let’s install ExpressJS framework.
Install ExpressJS
What is ExpressJS? It is,
"Fast, unopinionated, minimalist web framework for NodeJS. "
Straight from ExpressJS website. In other words, it is a framework for writing NodeJS applications. Using ExpressJS you can create a front end MVC application or a barebone REST API. I love it.
- Make a project directory.
mkdir express-api
- Change directory to express-api
cd express-api
- Run npm init : We are using node package manager to install and manage our packages. So what is npm init? It initializes your package with a “package.json” file. This file holds true metadata about your project. Read more on package.json @ https://www.npmjs.org/doc/files/package.json.html.
npm init
- By Running npm init it will ask you series of questions. Pretty much information about your project. Answer these questions and you should have a project.json file. Here is my package.json file,
- Run npm install express --save : This command will install expressJS in our project. By specifying --save at the end of this command it ensures that our package.json file is modified with the express dependency.
npm install express --save
In the image below you will notice express dependency saved automatically into our package.json file.
{
"dependencies": {"express": "^4.10.0"}
}
Now, open Sublime Text. Click “File | Open” and browse to the folder that contains “express-api” aka your project folder. Click on “express-api” and select “open”. You will see express enabled project in the IDE. This is what I see when all said and done.!
I am a foodie. I love good foods and therefore, in this series we will be creating a REST API for creating, updating and pretty much managing the entire recipes collection.
In Part II, we will look at setting up the backend in MongoDB. See you then.
Hi, I am Ritesh Patel. I live in a beautiful town surrounded by the mountains. C&O Canal is few miles away. State parks are only a distance away & bike trails galore. It is home sweet home Frederick, MD. A passionate developer. Love to cook. Enjoy playing "Bollywood Tunes" on my harmonica. Apart from that just a normal guy.