Node REST API - Part II
NoSQL Thingy!
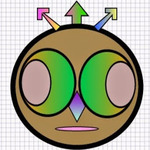
In Part I of this series I showed you how to setup development tools for writing a REST API. In this tutorial we will prepare MongoDB with some normalized collections for our API.
Few words on MongoDB. It is a documents database and one of the leading NoSQL databases. When I say documents database, I mean it stores documents with key-value pairs.
So what does a MongoDB document look like?
It’s a simple JSON document with some key-value pairs.
{
"_id": 1,
"name ": "Baked Ziti",
"title ": "Delicious Pasta made with marinara sauce & heavenly four cheese!",
"prep_time ": "10 minutes",
"total_time ": "40 minutes",
"difficulty ": "easy",
"recipe ": "This is the key that stores our entire recipe ",
"recipe_type ": "Italian",
"tag_words ": "Italian, Pasta, Easy Italian Recipe ",
"contributor ": "Bobby Flay ",
"stars": 5
}
Sure enough, I have some important metadata for a recipe. Name, title, preparation time, total time etc...This is the beauty about documents database. You can list all key value pairs in one big document. But wait, you might say I have a traditional RDBMS background. How would I setup this document with linked documents? Well, super easy. Let’s take a look at modeling this document into linked documents. In other words, say normalized documents.
We will create 3 different documents.
- RecipeTypes
- Contributors
- Recipes
Recipe Types Document
[
{
"_id": 1,
"recipe_type": "Italian"
},
{
"_id": 2,
"recipe_type": "Indian"
},
{
"_id": 3,
"recipe_type": "Thai"
},
{
"_id": 4,
"recipe_type": "Mexican"
}
]
Contributors Document
[
{
"_id": 1,
"contributor_name": "Ritesh Patel",
"account_status": "Active"
},
{
"_id": 2,
"contributor_name": "Bobby Flay",
"account_status": "Active"
}
]
Recipes Document
{
"_id": 1,
"name ": "Baked Ziti",
"title ": "Delicious Pasta made with marinara sauce & heavenly four cheese!",
"prep_time ": "10 minutes",
"total_time ": "40 minutes",
"difficulty ": "easy",
"recipe ": "This is the key that stores our entire recipe ",
"recipe_type ": 1,
"tag_words ": "Italian, Pasta, Easy Italian Recipe ",
"contributor ": 2,
"stars": 5
}
Nothing special here, right? I broke down a single Recipe document into 3 separate documents.
RecipeTypes: Stores various recipe types in a separate document and we assign Id to each recipe type which will be tied into our “Recipes” document.
Contributors: Stores all contributor accounts for our recipes collection.
Recipes: Our main recipes collection that will store all of our recipes. Pay attention to “recipe_type” and “contributor” keys. recipe_type is tied using the _id from recipes collection and contributor is tied using the _id from contributors collection.
Simple enough, huh?
Now let’s look at some very basic MongoDB commands to add and query the data. Of course we will call our database a “recipesdb”. Open up a terminal or a command line and type “mongod” at your terminal.
mongod
This will start MongoDB daemon process to handle data requests, manage data format and run background operations.
With our “mongod” daemon running, open up another terminal window and type in “mongo”.
mongo
This is our mongo cli. You will see MongoDB version and a simple message that says “connecting to test”.
Now let’s look at some mongo commands.
Command below will display a listing of databases in our MongoDB. Sure enough we don’t have our “recipesdb” in the listing yet.
show dbs
Command below will tell Mongo Cli to use “recipesdb”. Terminal will show a message “switching to db recipesdb”. Now run “show dbs” again. You’d think mongo will list our recipesdb, right? Not yet. Mongo will only create our “recipesdb” when initialized with at least one document. In other words we have to create a document to be stored in our database.
use db recipesdb
Insert Records
db.recipetypes.insert({
_id:1,
recipe_type: "Italian"
});
db.recipetypes.insert({
_id:2,
recipe_type: "Indian"
});
db.recipetypes.insert({
_id:3,
recipe_type: "Thai"
});
db.recipetypes.insert({
_id:4,
recipe_type: "Mexican"
});
Self explanatory! We are inserting four recipe types to our document. Our very first insert statement will implicitly create “recipesdb”, “recipetypes” collection and insert a record into recipetypes collection.
Read Records with - find()
Meet find(). One way to query MongoDB collection and view the data. find() also has a brother “findOne()” which filters the collection and targets data based on the condition passed in by the user.
db.recipetypes.find()
Command above will simply list records from recipetypes collection.
Filter Records with - findOne()
db.recipetypes.findOne({"recipe_type":"Indian"});
As you notice, we are filtering records by passing in a condition to findOne() function. We are specifically interested in viewing a recipe type of "Indian". Cool, right?
Update Data
Indian cuisine is all about spices, right? So let's update "Indian" recipe type to "Spicy Indian". It is super simple and easy to do so. Let me show you.
db.recipetypes.update(
{_id:2},
{
$set:{recipe_type:"Spicy Indian"}
});
Statement above tells Mongo to look for a record with _id:2 and then set recipe_type from "Indian" to "Spicy Indian". That's it. Don't believe me, well run this command in your Mongo Cli and you shall see it yourself :-)
db.recipetypes.find();
In Part III of this series we will review NodeJS. Pretty much a primer on NodeJS.
See you then!
Hi, I am Ritesh Patel. I live in a beautiful town surrounded by the mountains. C&O Canal is few miles away. State parks are only a distance away & bike trails galore. It is home sweet home Frederick, MD. A passionate developer. Love to cook. Enjoy playing "Bollywood Tunes" on my harmonica. Apart from that just a normal guy.