Node REST API - Part VIII
Log my calls!
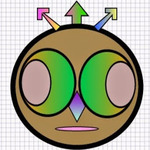
Kudos for staying with me through the entire series on Node REST API. This is the last and final tutorial in this series. I could have stopped this series at previous tutorial but I feel there is one more piece left and that is to integrate logging capabilities for our REST API. I personally would like to know if something has gone wrong in the code. Say, I’d be able to REST much better by logging my calls and everything else in the API.
Meet Winston!
No Winston is not my buddy, instead it is my REST API’s buddy. I love winston logger. First things first. Let’s install winston module. You have seen this before.
npm install winston --save
Command above will install winston dependency in our project and modify package.json with Winston dependency. Here is my package.json file.
{
"name": "express-api",
"version": "0.0.1",
"description": "ExpressJS REST API",
"main": "index.js",
"dependencies": {
"express": "^4.10.0",
"mongoose": "~3.6.13",
"winston": "^0.8.3"
},
"devDependencies": {},
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1"
},
"author": "Ritesh Patel",
"license": "ISC"
}
Setup Winston
We will create a simple utility module called logger using Winston. Let’s create a new folder called utils under express-api. Right click on express-api and add a New Folder utils. Under utils add a new file logger.js.
We also need a place to store these logs, right? We will use a file system to store Winston logs. Therefore, let’s create one more folder under express-api called logs.
When all said and done our project structure should be as below. Oh and winston will create a log file if one doesn't exist. Therefore, no need to manually create the winston.log file.
Now open up logger.js file. Cut and paste the code below into logger.js.
var winston = require('winston');
var config = {
levels:{
silly:0,
verbose:1,
info:2,
data:3,
warn:4,
debug:5,
error:6
},
colors:{
silly:'magenta',
verbose:'cyan',
info:'green',
data:'grey',
warn:'yellow',
debug:'blue',
error:'red'
}
};
var logger = new(winston.Logger)({
transports:[
new (winston.transports.Console)({
colorize:true
}),
new (winston.transports.File)({
filename:__dirname + '/../logs/winston.log'
})
],
colorize:true,
levels:config.levels,
colors:config.colors
});
module.exports = logger;
Let’s review this code. We require winston module. Next we colorize winston with logging levels and color codes. Look at various logging levels and color codes offered by winston. Pretty awesome, right? I love colors and therefore am in love with this logger.
Next we instantiate the logger. Pay attention to transports collection. We are asking winston to log in 2 different places.
- Node Console
- File System
With the file system we are also specifying winston to store log file under logs and name it winston.log. Straight forward, right?
Let’s call Winston!
Now let’s call Winston. Shall we?
Open up routes.js file in Sublime Text. We will log a very simple & informative message in our welcomeAPI function. We will import the logger module from utils.js file. Import means require.
var logger = require(__dirname + '/../utils/logger');
Now let’s log an informative message.
/**
* welcomeAPI()
* @Request GET
* Root API route message
*/
exports.welcomeAPI = function(req, res){
logger.log('info', 'Recipe API called!');
res.json({message:'Welcome to Recipe API!'});
}
Pay attention to the line below.
logger.log('info', 'Recipe API called!');
When a user or application makes a call to localhost:3000/api our logger will log a message in the log file as well as display the same message on the node console.
Let's test this using the REST client.
Sure enough, I made a call to the REST API. Now let’s examine node console. You have a server running, right? Yes, I am referring to your express server console.
Now, let’s see if Winston created a log file, and to my surprise it did. Here are the contents from my winston log file.
{"level":"info","message":"Recipe API called!","timestamp":"2014-11-17T17:41:18.704Z"}
Easy, huh?
Play with various log levels and colorize your node console :-)
Here are few sample javascript calls.
logger.log('error', 'Oooops! something went wrong!');
logger.log('warn', 'I am warning you!');
logger.log('silly', 'You silly!');
logger.log('data', 'I love data!');
...and you get the gist.
With this I conclude our “Node REST API” series. Hope you enjoyed learning with me. If you did then pen down a line or two. Would love to hear from you.
Oh and here is the link to entire project on GitHub. Enjoy!
See you again!
Hi, I am Ritesh Patel. I live in a beautiful town surrounded by the mountains. C&O Canal is few miles away. State parks are only a distance away & bike trails galore. It is home sweet home Frederick, MD. A passionate developer. Love to cook. Enjoy playing "Bollywood Tunes" on my harmonica. Apart from that just a normal guy.