Node REST API - Part III
Node Primer
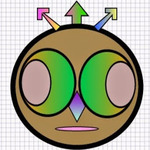
In Part II of this series we learned how to work with MongoDB. Before we dive into writing the REST API it is critical to review some basics on NodeJS. No, I am not going to bore you with yet another HelloWorld sampler, instead we will review what is important for writing a REST API.
I am assuming you have gone through the installation steps in Part I of this series. Sure you have. So let’s move on.
Browser Vs. NodeJS
When you think of JavaScript, first thing that comes to mind is a Browser, right? But Node JS is different. Sure it’s JavaScript, but without a browser. With browser you get a window, DOM, document & some.
In browser JavaScript do you know what happens if you forget to put “var” in front of a variable? Well, it goes straight into global namespace. In other words it pollutes your global namespace. In browser JavaScript how did you connect various functions and scripts? I say by including them using the script tag, right? Well, with Node comes the modules.
By default in NodeJS everything is local. If we need to access the Global scope then there is a special global object. How nice! Every script you run using Node is a process and a process has an id tied with it. You can easily view the process id by issuing the following command in a node console.
console.log(process.pid)
Modules / Exports / Require
In Node JS module is a king. You write modules, then you export and require these modules. Simple. Let me show you how easy it is to create and use a node module. As I said, I am a foodie. So let’s create a module that will teach us how to make a spicy pasta sauce. Shall we?
I will start with a new JavaScript file: pastaSauce.js. How obvious? Below are the contents of pastaSauce.js.
var pastaSauce = {
listIngredients:function(){
var ingredients = ['Ripe Tomatoes', 'Water (1 cup)', 'Oregno', 'Garlic', 'Salt', 'Sugar', 'Black Pepper', 'Fresh Basil', 'Red Crush Peppers'];
console.log('\n');
console.log("************************************");
console.log("Let's make a pasta sauce.\n");
console.log("Ingredients:")
console.log(ingredients.join());
console.log("************************************");
},
makeSauce:function(){
var process = [
'1) Saute garlic, oregno in 2 tablespoons of Olive oil',
'2) Puree ripe tomatoes in a blender',
'3) Add tomatoe puree to saute garlic',
'4) Add 1 cup water to the mixture',
'5) Add salt & sugar to your taste',
'6) Want a spicy sauce? Add red crush peppers to your taste',
'7) Let it simmer & then top it off with fresh basil leaves',
'8) Smells delicious!'
]
var i;
for(i=0; i < process.length; i++){
console.log(process[i]);
}
console.log('\n');
console.log("Now go eat, will ya?");
console.log('\n');
}
}
module.exports = pastaSauce;
Now let’s review this code. I am creating a literal object with few functions inside of this object: listIngredients() & makeSauce() respectively. Pay attention to the very last line of this code.
module.exports = pastaSauce;
I am exporting the entire object. In other words, I am exporting pastaSauce module.
Now, let’s create one more JS file call-recipe.js. Sure enough, we will call our pastaSauce module from call-recipe.js. In order to use pastaSauce module all we have to do is require our module in call-recipe.js.
Let’s review this code now.
//require pastaSauce module
var sauce = require('./pastaSauce');
//list ingredients
sauce.listIngredients();
//call makeSauce
sauce.makeSauce();
Code above makes the pastaSauce module available for use. Pay attention to the syntax here. Any modules within the same directory requires “./” in front of the module name. I don’t have to put “.js” extension in the require statement. Although I can, it won’t hurt. Next we list ingredients and then finally we make the sauce :-)
Now let’s execute these node scripts. Go to the terminal or a command line and type in,
node call-recipe.js
And voila! Now we know how to make a spicy pasta sauce!
Now that was simple, right? Let's review what we did.
- We created & exported a new module
- We imported this new module by using "require"
- We called the module methods to perform actions
Node http Module
We will be mainly using http core module for writing our REST API.
http - This module is responsible for Node JS HTTP server. Now let’s look at few of the methods offered by this module.
- http.createServer() - sure enough creates a new web server
- http.listen() - listens on a port of your choice.
- response.write() - sends a response to the client
- response.end() - sends / ends response body
Quick review of other modules
util - a utility module. It provides debugging capabilities.
querystring - used for serializing and deserializing the query string.
url - used for parsing and resolution of the urls.
fs - used for reading and writing files to the file system.
If you need additional capabilities then look no further. Go to Node Package Manager and find the package that will suit your needs.
Node Callbacks
It wouldn’t be fair if we talk about Node and ignore callbacks :-). So what are the callbacks? Just remember callbacks make Node asynchronous. In rather simple terms: We are making the pasta sauce. While the sauce is on the burner why not boil the pasta?
Now let's add one more method to our pastaSauce.js file. We will call this method boilPasta().
boilPasta:function(){
console.log('\n');
console.log('While sauce is being made...I will boil pasta');
console.log('Done boiling pasta, now waiting for the sauce');
console.log('\n');
}
Next, let's make minor modifications to introduce a timeout into recipe-call.js file.
//list ingredients
sauce.listIngredients();
//intentional timeout to demonstrate the callback pattern
setTimeout(sauce.makeSauce, 5000);
//boil pasta while we are waitng for sauce to be made
sauce.boilPasta();
In the code above pay attention to this particular line.
setTimeout(sauce.makeSauce, 5000);
I have intentionally introduced a 5 seconds timeout before calling the makeSauce() function. On next line I am calling boilPasta(). You will notice, boiling pasta doesn’t have to wait for the sauce to be ready. In other words, after calling setTimeout, node continues on to the next statement. When the 5 seconds timeout is completed, makeSauce() will be called, but by that time we are done boiling pasta.
Now running these changes into Node console I get,
Notice any changes? I see that we boiled pasta before listing the recipe steps for making a sauce.
Let’s consider one more scenario. You are given a task to read a 10GB csv file with some accounting data. While this huge file is being processed you are asked to read 5 smaller csv files and provide the user with an output.
Without callbacks, you would have to wait for the 10GB file to be processed. In other words, that 10GB file is blocking you from performing other operations. With Node callbacks you do not have to wait. A callback supplied to the readFile will process the response when it’s available while the program carries on with performing other tasks.
We will be using the callback pattern in writing the REST API. Therefore it is critical you understand how the callback pattern works with Node JS.
Since most of the formalities out of the way, finally we can start writing our REST API.
In Part IV of this tutorial series we will learn about ExpressJS and few additional tools .
See you then!
Hi, I am Ritesh Patel. I live in a beautiful town surrounded by the mountains. C&O Canal is few miles away. State parks are only a distance away & bike trails galore. It is home sweet home Frederick, MD. A passionate developer. Love to cook. Enjoy playing "Bollywood Tunes" on my harmonica. Apart from that just a normal guy.