Node REST API - Part IV
Full Speed Express!
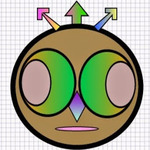
Stage is almost set. In previous tutorials we learned how to set up our environment, worked with MongoDB & also learned basics about NodeJS. Now let’s look at ExpressJS - A Framework for writing REST API with NodeJS. Express JS documentation is pretty awesome. Therefore, no need to paraphrase the documentation instead, I will review few important topics that we will be using for the REST API.
App Express
Express JS is based on Node. We learned in our previous tutorial about Node modules, how to export a module and require a module for use. And so, you guessed it right! For any express enabled application we must require express module.
var express = require('express');
Then we instantiate our express app with a single liner.
var app = express();
And now you have an instance of express application.
Express Middleware
Middleware is a function with access to request & response objects. Additionally, it has access to the next() middleware, in other words next() will move the execution to the next middleware in line. I know it’s confusing and therefore let’s look at a simple example.
Moving back to our recipe example. Let’s say we want to make a Baked Ziti. Each time we wish to make a Baked Ziti, we have to boil pasta, right? Without pasta there is no ziti. Therefore, each attempt to make a baked ziti must pass through a process of boiling pasta and then draining pasta. In other words boiling pasta and draining pasta are our middlewares. Let’s create an express app named bakedZiti.
var express = require('express');
var bakedZiti = express();
//now let’s introduce middleware
bakedZiti.use(function(request, response, next){
//deal with request object (if required)
var chefName = request.param.chefname;
//deal with response (if required)
//middleware logic
console.log('Boil Pasta');
//move on to next middleware
next();
});
//now execution moves on to this middleware
bakedZiti.use(function(request, response, next){
console.log('Drain Pasta');
next();
});
Notice how our application logic falls into the first middleware which will get chef’s name from the request object, then boil pasta and move on to the next middleware which is to drain pasta. You can have as many middlewares as you wish.
Use middlewares to manipulate request and response objects, set cookies, perform authentication logic etc.. Let’s put it this way. Middlewares rule in ExpressJS similar to how modules rule in NodeJS.
Later on we will implement a 3rd party middleware to enable logging in our REST API.
Error Handlers
Let’s face it. Error handlers are a cornerstone of any application: a well written application. A good developer must handle errors gracefully and since we are awesome developers we will do the same. In ExpressJS it is recommended to put error handlers at the very bottom after the app.use() calls. Error handler in ExpressJS looks something like this.
app.use(function(err, req, res, next){
var status = res.status || 500;
res.status(status);
res.render('error', {
error:err.stack,
message:'Oooops! Something went wrong - ' + err.message
});
});
Error handler function takes four parameters.
- err - Error object
- req - Request object
- res - Response object
- next - Next middleware to continue execution
In the code above we are retrieving response status and formulating a JSON response with error stack and an error message. You can also write error messages to log files with logger middlewares.
Tools to test REST API
We are going to write a REST API. It will be a barebone REST API without any front-end attached to it. How do you test your REST API? Well, there are multiple tools available to view REST communication. I personally like Chrome’s REST client extension. Pretty slick. It allows you to perform Get, Post, Put, Delete operations against your REST API. Additionally, you can view the entire communication, inspect request and response objects, confirm the type of response a REST call should send and so on. Here is what the REST client looks like.
You can also use cURL (curl) to test the REST APIs. cURL is more of a command line tool.
A very simple get call using cURL looks like this,
curl -H "Accept:application/json" http://localhost:3000/api
Pick your poison, right?
I absolutely salute your patience and willingness to read through these tutorials. It was definitely some grunt work. Finally, in Part V of this series we will dive into REST API using these tools. See you then.
Hi, I am Ritesh Patel. I live in a beautiful town surrounded by the mountains. C&O Canal is few miles away. State parks are only a distance away & bike trails galore. It is home sweet home Frederick, MD. A passionate developer. Love to cook. Enjoy playing "Bollywood Tunes" on my harmonica. Apart from that just a normal guy.