Node REST API - Part V
Enter REST API
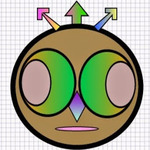
Geez! That was a lot of grunt work. Finally we are ready to dive in and start writing the REST API using ExpressJS, NodeJS & MongoDB. First things first. Let’s add index.js file to our project.
Open “express-api” project in Sublime Text. Right click on the project and click add file. Save file as “index.js”. This file will be the entry point to our REST API.
Let’s look at the outline of index.js.
- Application dependencies
- Instantiate express application
- Set application specific variables / paths
- Setup Mongoose ODM
- Define error handler
- Define api routes
- Create node server
- Export application
Application Dependencies
Of course we need express module. Along with express module we will also use core http module, path module and a body-parser module. Code below shows how we import these modules into our application. Remember node export and require module in Part III?
var express = require('express');
var http = require('http');
var path = require('path');
var bodyParser = require('body-parser');
Instantiate Express App
Now let’s instantiate express app and store the application reference in a local variable called app.
var app = express();
Application setup
With application handle in place, let’s add some configuration to the app. We will store API routes and models in a separate folder called “api”. Therefore let’s add “api”folder to our project. Right click on the project in Sublime Text. Add New folder called "api". Now we need to inform our app about this new folder.
app.set('api', path.join(__dirname, '/app/api'));
Next let’s add the server port. I see the node world using port 3000 for the server and therefore we will do the same.
app.set('port', process.env.PORT || 3000);
Let’s configure body-parser module. Our REST API calls will return JSON responses. We need a way to handle JSON responses. body-parser will help us accomplish that.
app.use(bodyParser.urlencoded({extended:true}));
app.use(bodyParser.json());
Mongoose / MongoDB configuration
What is Mongoose? Mongoose is a mongodb elegant object modeling for node js. Here is the definition straight from Mongoose website.
Mongoose provides a straightforward, schema-based solution to modeling your application data and includes built-in type casting, validation, query building, business logic hooks and more, out of the box.
Let’s add Mongoose dependency to our project. I will modify package.json as below. If you remember in Part I of this series we added express dependency to our project.json file. We will follow the same steps and add Mongoose to our project.
Add mongoose to package.json dependencies as below.
"dependencies": {
"express": "^4.10.0",
"mongoose": "~3.6.13"
}
Now open up terminal and go to “express-api” folder.
cd express-api
Run npm install at the terminal. This command will look at our dependencies in the package.json and add missing dependencies to our project. In this case, express has already been added and so it will add mongoose to our project.
npm install
In next tutorial we will look at Mongoose models in detail. So how do you connect to MongoDB using Mongoose?
//database connection
var mongoose = require('mongoose');//mongoose orm
mongoose.connect('mongodb://@localhost:27017/recipesdb', {safe:true});
Now of course I have a local mongo db running on my machine.If you followed Part I of this series then you should have a mongo running locally as well.
Error Handlers
As mentioned in Part IV it is recommended to add error handlers after app.use() commands. Error handlers are a cornerstone for any well written application.I say, we are awesome developers therefore we will do the same. Add a simple error handler. After all, we want to handle errors gracefully right?
//error handlers
app.use(function(err, req, res, next){
res.status(res.status || 500);
res.render('Recipe API Error', {
message:err.message,
error:err.stack
});
});
API Routes
Pay attention to API routes. Routes are the endpoints in our REST API. What is a route? It is simply a URL and then some. When an application recognizes our API route, it will call a route function. This route function will process the request and return a JSON response. Let’s look at some of the routes for our REST API.
/** API Routes **/
//router middleware
var router = express.Router();
We will use built-in express router for our application. Our routes module will be implemented in routes.js under api folder. For now let’s inform our application about the routes module.
//routes implementation modules
var routes = require('./api/routes');
Next let’s add routes for our REST API.
router.get('/api', routes.welcomeAPI);//api end point
How exactly you use these routes? Let's look at the first route '/api'. If I am making a call to localhost:3000/api then eventually our application will call the route function tied with this route. Which is routes.welcomeAPI. We will learn more about route functions in the routes tutorial.
Finally, let’s use this router.
//use router middleware
app.use('/', router);
Create a Node Server
We are almost at the end of our index.js file. We configured our express application, mongoose ODM, defined error handlers & routes. Now we need to create our Node server or else how would you access our delicious recipes API?
Let’s create a server
http.createServer(app).listen(app.get('port'), function(){
console.log('Express Server Running!');
});
In the code above we are creating a server on a port 3000 and listening for the requests. Every time we run this server a message “Express Server Running” is displayed in our node console.
Export App
Finally we export our application.
exports = module.exports = app;
Complete index.js
Here is what our index.js looks like.
'use strict';
//required dependencies (including 3rd party middlewares)
var express = require('express');
var http = require('http');
var path = require('path');
var bodyParser = require('body-parser');
//instantiate express application
var app = express();
//set api directory
app.set('api', path.join(__dirname, '/app/api'));
//set node http server port
app.set('port', process.env.PORT || 3000);
//configure body-parser for JSON
app.use(bodyParser.urlencoded({extended:true}));
app.use(bodyParser.json());
//database connection
var mongoose = require('mongoose');//mongoose orm
mongoose.connect('mongodb://@localhost:27017/recipesdb', {safe:true});
//error handlers
app.use(function(err, req, res, next){
res.status(res.status || 500);
res.render('Recipe API Error', {
message:err.message,
error:err.stack
});
});
/** API Routes **/
//router middleware
var router = express.Router();
//routes implementation modules
var routes = require('./api/routes');
router.get('/api', routes.welcomeAPI);//api end point
//use router middleware
app.use('/', router);
//create server & listen on port 3000
http.createServer(app).listen(app.get('port'), function(){
console.log('Express Server Running!');
});
//export app
exports = module.exports = app;
We are not ready to start the node server yet and here is why. Pay attention to API route in our index.js file.
router.get('/api', routes.welcomeAPI);//api end point
Where is the function routes.welcomeAPI defined? Nowhere right? Don't worry we will but not before looking at Mongoose Modeling.
In the Part VI we will look at setting up mongoose models.
See you then.
Hi, I am Ritesh Patel. I live in a beautiful town surrounded by the mountains. C&O Canal is few miles away. State parks are only a distance away & bike trails galore. It is home sweet home Frederick, MD. A passionate developer. Love to cook. Enjoy playing "Bollywood Tunes" on my harmonica. Apart from that just a normal guy.